We are about to switch to a new forum software. Until then we have removed the registration on this forum.
Hi. I'm doing this videoplayer but I need to see the progress of the video and be able to change it or make jumps clicking on it. I tried to update the internal value of the slider in an external function but the script got in a loop. It's possible to make this with a P5 slider?
Answers
I'm stuck with the same problem.. Have You found a solution for using a P5 slider as a progress bar?
Here the code of my sketch:
I want to be able to navigate inside a music track modifying the position of the slider but when the event
ACTION_PRESSED
is firedtheEvent.getController().getValue();
doesn't contain the new value of the slider that i selected through my action but the old value( the value that indicate where the music track is arrived).. Any help? Thanks! :-SHi, following up on the code that you posted, I came up with the following 2 suggestions. Controlling the value of a slider here is indeed a bit tricky due to how mouse events and value changes are handled. Suggestion 1 below uses a Slider to achieve the effect you are looking for which works alright for a mouse pressed. when dragging the slider though the value is not set as expected due to the original implementation of the slider. Therefore, have a look at Suggestion 2 which uses a custom Controller called ProgressBar.
Suggestion 1
Suggestion 2 using a custom Controller class.
Hello,
I decided to put here the same problem I'm having this old post due to be basing myself in the previous example.
I'm not able to run when I put the objects to be created in a second window (extends Applets).
When I try to run gives the following error as print below:
Any idea how to solve this?
Below the code I'm doing the test:
//Program Menu ( Call Video )
//Program PlayVideo
Note: If you leave this unique program, works OK.
Thank you,
Hello,
I could make it work with another example that is up, I'll post here, it may be useful to someone else.
//Program Menu
//Program Play Video
Thank you,
Hello gumo
Yes, the code above does this, you can append (the end button) with this code.
As you "click" on the bar, the movie moves to that point ...
I just converted the time to min. I think you need to see some rule for this.
Any questions I can send a more complete code ...
Nice! --and more code would be great Jose --thanks!
Hello grumo,
Sorry for the delay, I was not looking at the email these days ...
Below is the code, the first part of the program creates a Menu, which according to the example you can add other new links.
Below is the "player" program, the examples I took here in the forum and adapted according to my need, you can adapt in the best way for you.
You need to grab some images for the Play, Pause, End, and Start Menu button too, of course ... put it in the "date" folder ....
I have not commented every part of the program, if you need help in this regard, return that I can improve this ...
Regards,
//First part of the Program (Create Menu)
//Second Part of the Program (PLayer)
I forgot a detail, the second part of the program being in extended class, need to add the path where the images are, does not recognize that it is in the folder "data" ...
Put this variable before setup:
Change this line where you upload the images
.setImages(loadImage(PATH+"/play.png"), loadImage(PATH+"/play.png"), loadImage(PATH+"/play.png")) //need picture at data
Print how was the example
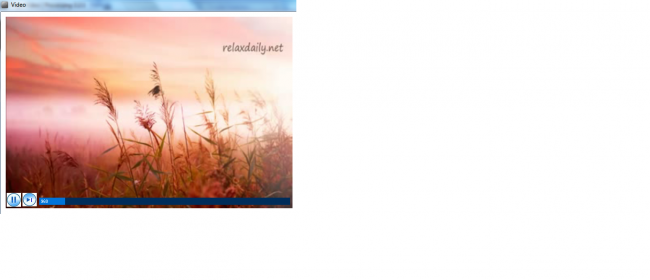
Has anyone got this to work - it does not work for me - maybe a problem with declaring setup() twice?
I haven't tested this, but if I understand right
setup()
is not declared twice.setup()
is a function of the main sketchPlayVideo.setup()
is a method of PlayVideoThe progress bar works fine but I am having trouble getting it to update as the video plays. Can someone help me?
`
@Izzy280
Please format your code. Edit your post (gear on top right side of any of your posts), select your code and hit ctrl+o. Leave an empty line above and below your block of code. Details here: https://forum.processing.org/two/discussion/15473/readme-how-to-format-code-and-text
I suggest you create a new post and link this post there. This will allow you to receive notifications when somebody comments on your posts, as you will be the owner of that post. Nevertheless, adding this link to your post will provide valuable info to address your question.
Please also provide more insight about your problem:
EDIT*******
CONTINUE discussion at https://forum.processing.org/two/discussion/25606/video-tracking-for-progress-bar-using-movie-and-controlp5#latest
Kf
No problem. Thank you.