We are about to switch to a new forum software. Until then we have removed the registration on this forum.
Hello
I'm looking for a way to code a Frosted Glass surface on top of a P3D render in processing. I guess it will use some glsl shader and I've played a bit with them but didn't find a way to constrain the shader to a surface.
float alpha = 0;
void setup() {
size(640, 360, P3D);
rectMode(CENTER);
}
void draw() {
background(255, 128, 128);
pushMatrix();
fill(255);
translate(width/2, height/2, -30);
rotateY(alpha);
rotateX(alpha);
box(150);
popMatrix();
pushMatrix();
fill(255, 64);
translate(width/2, height/2, 120);
rect(0, 0, 200, 100);
popMatrix();
alpha+= 0.01;
}
what do you think would be the best way to make this rectangle blur what is behind it ?
cheers
Answers
Could you copy an area of the screen into a small rectangular PGraphics and then run the shader on the PGraphics?
I believe there are some "frosted glass" shaders listed on shadertoy -- but I am no shader expert.
That's the kind of idea I'm looking for :)
But is it possible to render the view of "a" camera into that rectangle PGraphics so that I could move the rectangle ?
Sure -- rather than
image(pg,0,0)
you cancopy(pg, [camera geometry])
:BTW, you mentioned both frosted glass and a moving camera. On the off-chance you are trying to create a looking-glass effect, these examples might be interesting:
- https://forum.processing.org/two/discussion/comment/76980/#Comment_76980 - https://forum.processing.org/two/discussion/comment/108038/#Comment_108038
Really nice but I'm looking for something like that https://codepen.io/jonitrythall/pen/GJQBOp
to be able to draw a frosted glass plane inside a 3D scene
@phoebus -- huh.
I just replaced a few lines at the beginning of the sketch setup to turn the two images into an unblurred and blurred version of the same image. Without any other changes it seemed like it was doing exactly what you wanted....
See PImage, PImage.copy(), and PImage.blur(): https://processing.org/reference/PImage_filter_.html
Of course, a shader will perform much better! But if you are only blurring the background once and not every frame then that performance probably doesn't matter.
Also, possibly related on the GLSL side:
this code does work as "Frosted Glass" for a certain image
But I don't get how I can have it working here
Ah, that second approach is less flexible but much simpler if you just want to wash a 3D scene with a partially transparent white rectangle.
When you say "have it working" I assume that you mean there is a problem because you are losing the lines on your cube? you need to add this to setup:
That should create a brightening effect without the line loss:
For more on OpenGL hints, see the JavaDoc API:
(it's not that easy have to interact with fellow creative coder only by forum and never in person :S)
So, I have this Code
it looks like that :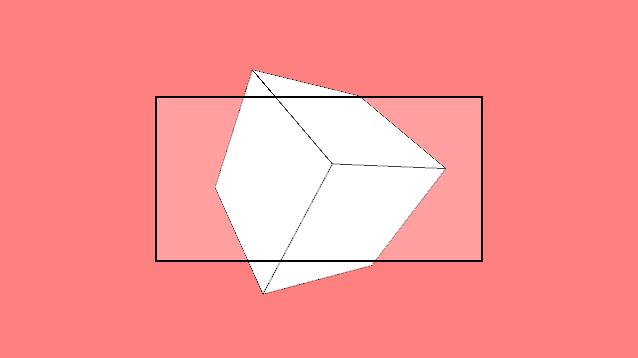
and I would like it to look like that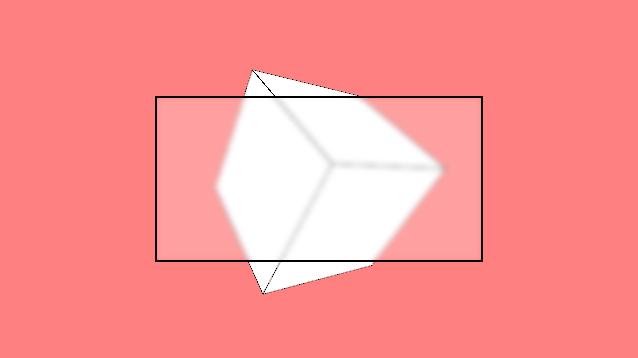
i think you are searching for something like this:
processing code:
glsl code:
you can change the statement if to modify the blur rectangle.
hope this help you :)
spot on @pietroLama !
so here it is; exactly what I was looking for
for the sketch and for the glsl file
and a special thank to @jeremydouglass for the shader free tricks
i suggest you to modify the code like this.
glsl:
processing:
great !
then I guess we can have radX and radY, or even a way to set the frosted rectangle with parameters like (x, y, w, h)...
thank !
@pietroLama
would this thing work on P5.js ?
So this seems to have already a good solution, nice work!
Sometimes controling the LOD level of the texture is enough, maybe some1 find it interessting i leave it here:
Interesting. Given that the demo is blurring the whole image and not just the rect area, how would this actually be applied in practice to create the desired "frosted glass" effect? Would you apply the shader just to one image crop area, then layer the rect on top of that?
i updated the scetch, replaced the image and made the surface bigger.
this is what i see, can you confirm ?
So i running into strange bugs, means : - time to stop here.
I was only a sketchy idea, that needs some time and knowledge to develop it's potential. : )
Nevermind
Their is also an lib for filters, found it again, searching trough this forum.
https://github.com/cansik/processing-postfx
and as i said the current solution, seems to work.
i was only flyby this forum ....
It does look like an interesting approach. The updated version is much closer, although if you zoom in you can see the artifacts spilling out at the edges of the rect.
My guess would be that the best (and most intuitive) way to do this is to run the shader on a PImage or PGraphics -- not on the whole screen area. But I don't really know anything about shaders. Then a moving glass pane of any geometry is implemented by changing the buffer where you run the shader. But: I don't really know much about shaders.
thank you for respose. confirmed. An PImage "pre render" via second PGraphics Method at the size of the actual rectangle would be the best.
I also don't know how to do it :)