We are about to switch to a new forum software. Until then we have removed the registration on this forum.
I tested this code with other frameworks/engines It works fine.
In processing with different images/videos etc. you can see a line going through the screen
I guess, it's the default texture mode. Maybe a user already got a workaround
Any help is much appreciated.
Copy +Paste press Play
//Vertex
String[] vertSource={"#if __VERSION__ >= 150" //check backward compatible /mobile
, "#define attribute in"
, "#define varying out"
, "#endif"
, "#ifdef GL_ES"
, "precision mediump float;"
, "precision mediump int;"
, "#endif"
//attribute
, "in vec4 position;"
//uniforms
, "uniform mat3 Ry;"
, "uniform float pitch;"
//varying
, "out vec3 refDir;"
, "void main() {"
, "gl_Position = vec4(position.xy,0, 1.);"
//apply Rotation Matix
, "refDir = Ry*vec4(position.xy,1.,0).xyz;"
, "}"
};
//Fragment
String[] fragSource={"#if __VERSION__ >= 150" //check backward compatible /mobile
, "#define attribute in"
, "#define varying out"
, "#define texture(a,b) texture2D(a,b)"
, "#endif"
, "#ifdef GL_ES"
, "precision mediump float;"
, "precision mediump int;"
, "#endif"
//attribute
, "in vec3 refDir;"
//uniforms
, "uniform sampler2D envmap;"
//varying
, "out vec4 fragColor;"
, "#define PI 3.14159273"
, "void main () {"
//map texture to sphere: <a href="https://github.com/mrdoob/three.js/issues/1621" target="_blank" rel="nofollow">https://github.com/mrdoob/three.js/issues/1621</a>
, "float lat = atan(refDir.z,refDir.x);"
, "float lon = acos(refDir.y/length(refDir));"
, "fragColor = texture(envmap,vec2(.5+lat/(2.0*PI),lon/PI));"
, "}"
};
PShader shader;
PImage img;
PMatrix3D rotY = new PMatrix3D();
void setup() {
//setup scene
size(640, 360, P3D);
//load frag/vert Source
shader=new PShader(this, vertSource, fragSource);
//load equirectangular Image
//https://www.flickr.com/search/?l=commderiv&q=equirectangular
String https="https://"; //processing forum resolve links automatically - workaround to avoid it
img = loadImage(https+"c1.staticflickr.com/8/7178/7019373941_c1f2402f06_h.jpg");
}
void draw() {
//map mouse to a range form - to
float p=map(mouseX, 0, width, HALF_PI, -PI);
//uncomment for 360 rotation
//p=(float)millis()*.001f;
//rotation to mouse
float s=sin(p), c=cos(p);
//uniform Rotation Matrix
shader.set("Ry", rotY, true);
//reset to NULL
rotY.reset();
//Rotation (pitch) "http://planning.cs.uiuc.edu/node102.html/
rotY.apply(
c, 0, s, 0,
0, 1, 0, 0,
-s, 0, c, 0,
0, 0, 0, 1);
//clear
background(0);
//uniform texture image
shader.set("envmap", img);
//apply filter to scene
filter(shader);
}
Answers
How i suspected the error occurs in the wrorg texture filtering mode, switch to
((PGraphicsOpenGL)g).textureSampling(2); //nearest
fixed it.
hi nabr, found this by following a link someone posted here:
https://forum.processing.org/two/discussion/21909/how-to-change-the-textures-of-a-cubemapshader#latest
got some errormessages about non-varying refDir and fragColor. after defining these as varying variables, it seems to work to fine for me, for now
Hello @HBo
Your system ?
pc win7 64 bit processing 3.0.1
one question: when I comment hint(DISABLE_OPTIMIZED_STROKE), there appears a seam in the reflection -->
@ everybody do you know how to fix it? (please don't pay attention to the wrong relation of environment - reflection. i only need to get rid of that seam on the sphere)
@HBo: Please share your source. So i can catch up. I curretly bussy with my own stuff. when i setup the processing with a sphere already 10mins pass. And it's not garanted that i fix the problem.
So i can easily copy paste and start with debug. (You can also provide a zip file, would be also nice.)
@HBo This is not 100%, but from my experience
you look INSIDE the sphere. AND i don't know how to fix it. Something wrong with the normals. they should be flipped. And pointing always towards the camera
For Testing:
here's the code:
when you replace the sphere with the bunny, you also can see easily see its seams (e.g. at the green areas):
thank you in advance!
@HBo -- For that specific kind of projection you need a 360 panoramic image whose far left and right sides match up. Then there is no seam. For example, any of these images would work: https://www.google.com/search?q=360+panoramic+image&tbm=isch
Ah. I see that
envmap
is the correct type of image: I was wrong thinking that was the cause.@HBo i have to pass for today, but i will catch up soon. I think the default camara in processing have not the "right" settings, - that fits this task.
@jeremydouglass. No
Same shader code, accept for the cam.
https://cdn.rawgit.com/tolkanabroski/coding/master/regl/360/index.html
Here is a good example how to show the normals of a mesh. The outlines on the bunny comes from wrong normal direction.
https://github.com/codeanticode/pshader-experiments/tree/master/SphereSubdivGS
i flip them here
"vec3 eye = normalize(position.xyz-modelviewInv[3].xyz/modelviewInv[3].w);"
so i have a custom situation and still the default camera.
@HBO i would love to fix it. But their no chance currently. Here is a good old but gold tutorial how camera works.
http://duriansoftware.com/joe/An-intro-to-modern-OpenGL.-Chapter-3:-3D-transformation-and-projection.html
edit: @HBo https://processing.org/reference/normal_.html
first we shold pass a version i think this would solve the varying out problem
new String[]{"#version 150 \n"
https://github.com/processing/processing/blob/master/core/src/processing/opengl/PGL.java#L1912
second the problem is that the normal attribe is predefined and wrong in this situation:
so you have to overwrite it: https://github.com/processing/processing/wiki/Advanced-OpenGL#processing-3x
@jeremydouglass
http://www.reindelsoftware.com/Documents/Mapping/Mapping.html
edit:
@HBo interessting effects !works only on a processing sphere
+ "refDir = reflect(eye,vec3(normal.xy,1.-normal.z));"
and flip the image
+ "refDir = reflect(-eye,-vec3(normal.xy,1.-normal.z));"
wow! thanks for your effort. I'll have a look at it tomorrow
@nabr no seam on imported meshsphere created in rhino 3d: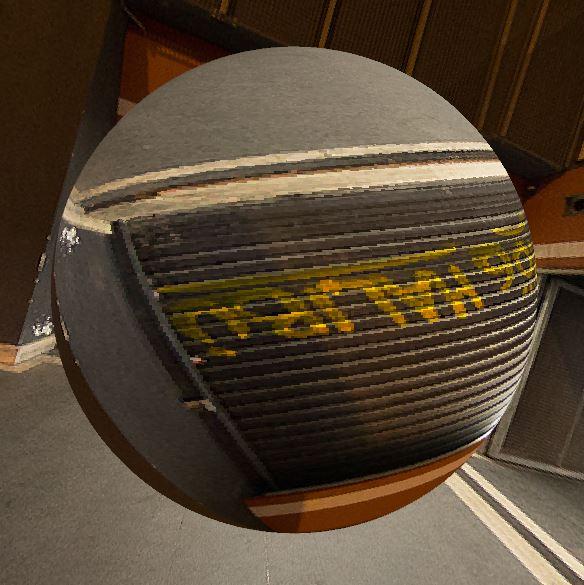
@HBo
Okay i know now, its my mistake:
=>i have a spherical projection of a equirectangular image
=>i run the same code to map in back from equirectangular image to spherical projection
=>i should map it from spherical projection to spherical projection or better : from World Space To Spherical
Rihno 3D: bunnyshader vertexshader replace refDir
****OR**** pure glas: bunnyshader fragshader replace fragColor
+ "fragColor = texture(envmap,vec2( .5+asin(refDir.x/PI) ,.5+ asin(refDir.y/PI) )) ;"
https://www.mvps.org/directx/articles/spheremap.htm
Full code comes tomorrow!
@nabr yes, there should be made some changes with spherical projection. the reflection an a planar (very low-res) meshplane looks like this:
i'm curious to see it...
@HBo
i dont really understand what is going on here i did a code clean up also read from forum post. this is what i got so far. I also out of time. Keep me posted with news:
@ nabr nice code! after replacing refDir as you suggested, there aren't any seams no longer. for a quick test, i loaded this simple meshterrain :
@HBo yeah unnecessary code for rotation
you dont really need a *.obj for a simple model if you got shaders :)
http://www.ozone3d.net/tutorials/vertex_displacement_mapping.php
@nabr yes i know the power of shaders. but i also know, how much more it still takes for me, until i see something on screen or even understand, what i'm doing. i'll deal with that, when i get in trouble with the framerate
Yes, i have a copy paste bug like everywhere
this is wrong
rotateZ(cos(t)*.5+.5);
explanation
EDIT no i was wrong (maybe) I have to rotate the camera! camera(sin(),cos()..... ) !
@HBo i hope, it's okay i use your test_terrain.obj on my >github < it's public. When not: PN me and i will take it down.
@ nabr no problem :)