We are about to switch to a new forum software. Until then we have removed the registration on this forum.
I am currently working on a game where you shoot stuff down in space and I cant figure out how to check if the bullets are hitting the targets heres the code
ArrayList <Bullet> bullets;
float numOfStars = 500;
PImage spaceShip;
float [] x = new float[int(numOfStars)];
float [] y = new float[int(numOfStars)];
float [] speed = new float[int(numOfStars)];
float [] bullet;
//Bullet = new Bullet();
float bulletSpeed;
boolean Bullet;
void setup() {
bullets = new ArrayList();
fullScreen();
//size(500, 400);
background(0);
stroke(255);
spaceShip = loadImage("Spaceship2.png");
noCursor();
int i = 0;
while (i < numOfStars) {
x[i]= random(0, width);
y[i] = random(0, height);
speed[i] = random(1, 5);
i = i +1;
}
}
void draw() {
background(0);
removeToLimit(100);
moveAll();
displayAll();
//bulletSpeed = bulletSpeed +5;
//draw triangle
//triangle(mouseX,mouseY-6,mouseX +20, mouseY,mouseX, mouseY +6);
//rotate(radians(180));{
image(spaceShip, mouseX-10, mouseY);
//}
int i = 0;
while (i < numOfStars) {
float co = map(speed[i], 1, 5, 100, 255);
stroke(co);
strokeWeight(speed[i]);
point(x[i], y[i]);
x[i] = x[i] -speed[i]/ 2;
if (x[i] < 0) {
x[i] = width;
}
i = i+5;
}
//if (mousePressed == true) {
// Bullet = true;
//}
// if (Bullet == true) {
// rect(mouseX -10 +bulletSpeed, mouseY, 40,10);
// fill(#03FC3E);
// }
}
class Bullet//bullet class
{
float x;
float y;
float speed;
Bullet(float tx, float ty)
{
x = tx;
y = ty;
}
void display()
{
rect(x,y+20, 40,10);
rect(x,y+80,40,10);
fill(#03FC3E);
//stroke(255);
//point(x,y);
}
void move()
{
x+= 20;
}
}
void removeToLimit(int maxLength)
{
while(bullets.size() > maxLength)
{
bullets.remove(0);
}
}
void moveAll()
{
for(Bullet temp : bullets)
{
temp.move();
}
}
void displayAll()
{
for(Bullet temp : bullets)
{
temp.display();
}
}
void mousePressed()//add a new bullet if mouse is clicked
{
Bullet temp = new Bullet(mouseX,mouseY);
bullets.add(temp);
}
Answers
edit post, highlight code, press ctrl-o to format.
circular collision detection is probably easiest. check distance between bullet and object is less than the sum of radius of both. but we'll know more when you format your code because it's currently unreadable.
I am really new to processing, can you write the code for me?
Please check this:
https://forum.processing.org/two/discussion/comment/90483/#Comment_90483
https://forum.processing.org/two/discussion/comment/89965/#Comment_89965
Kf
I dont get it... I have seen a lot of posts but still dont get it
@Kang01 --
In that case it sounds like you need a step by step tutorial in collision detection, with a slow, simple explanation for beginners and lots of working examples.
Here you go:
I have watched plenty of tutorials, and none of them helps
I still dont get it
Check this video: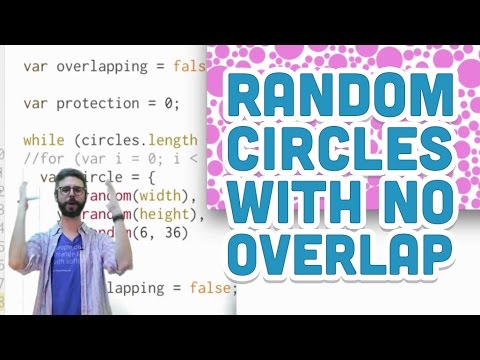
The important thing here is that the technique to avoid shape overlapping and to check for collision interaction is the same. If you check the first link in my previous post, I explained there how the algorithm works.
Kf
I just dont know how to check if two classes are colliding and I cant find anyone explaining it
Hello,
some advice:
house cleaning
you need to do regular house cleaning.
Throw those aways:
auto-format your code
Use ctrl-t to auto-format your code
order of things
I would recommend to order your code in a way that nothing comes behind the class or the classes. At the moment you have functions before and after the class.
Class Star
you could make a
class Star
similar toBullet
with a new ArrayList stars(
numOfStars
should be int, not float btw.)Collision
regarding your collision: There are no asteroids. Do you want to shoot the stars? That would be wrong technically since they are light years away.
They are just the background.
Asteroids would look cooler. Make a
class Asteroid
.Collision takes now place with the stars anyway in function
moveAll()
.You need to loop over all bullets and for each over all stars to check if they are close. I made a new method in the Bullet class named
isClose
. It is usingdist()
.This:
return dist(x, y, x1, y1)<33;
just means: return true when condition is true, otherwise return false; the boolean result of the comparison<
is immediately returned.Best, Chrisir ;-)
P.S.
Sorry, kfrajer, I haven't seen your post, we wrote at the same time but you were faster.
Thank you it is doing just what I wanted it to do!
No its not done yet I will ad some space invaders :)
in your function
removeToLimit()
you need a backward for-loop to
remove()
bullets if they are!isAlive
or when they left the screen (x>width+110
or so)So what do i write?
IN removeToLimit PLEASE:
like
not tested.
especially
i>=0
might be wrong.By the way
!
means not (logical not, which is inverting the value of a boolean)Thanks
Is there any way I can check for collision between the mouse and the enemies?
Use mouseX, mouseY in moveAll
What syntax?
dist
Show your attempt
I am, I am just really new
Sure
Collision between mouse and enemies
So loop over enemies and compare with mouse
Uae dist
As shown
Well, I dont get it just dont bother I will just give up
I posted your solution
Which line numbers are relevant?
Don't quit so soon, kid.
Thanks for your help, but I can't figure it out, I am just gonna try something new dont bother
This checks all stars against mouse