We are about to switch to a new forum software. Until then we have removed the registration on this forum.
/**
* Three-dimensional tree
* by Atoro
* www.youtube.com/channel/UCHzo-C2Ug4Ofp0CqY82qswA/videos
* www.facebook.com/BlankNewWorld
*
* A very simple example of
* generating a tree in three-dimensional space
* using recursive functions.
* (Processing 2.1)
*
* rt3d0001
* 2013-12-11
*/
float len = 125; // These two numbers define
int knees = 10; // the height of the tree
int recursions = 5; // Larger number corresponds to more branched tree
int branches;
void setup() {
size(800, 600, P3D);
smooth();
stroke(0); // Color of the tree
noiseSeed(119); // Different number -- different tree
}
void draw() {
background(255);
translate(width/2.0, height, -100); // Position of the tree
rotateY(frameCount / 100.0); // Rotation speed
branches = 0;
drawBranch(len, 0, 0, 0, knees, recursions); // Draw the tree
println("Branches in tree:", branches);
}
// *** Recursive function
void drawBranch(float le, float bx, float by, float bz, int kn, int rec) {
rec--;
if (rec >= 0) {
branches++;
pushMatrix();
translate(bx, by, bz);
float rxyz[][] = new float[kn][4];
rxyz[0][0] = map(noise(rec+branches, 0), 0, 1, 0, le);
rxyz[0][1] = 0;
rxyz[0][2] = 0;
rxyz[0][3] = 0;
float an1, an2;
for (int i = 1; i < rxyz.length; i++) {
/* x=Rsin(an1)cos(an2), y=Rsin(an1)sin(an2), z=Rcos(an1)
More info: http://en.wikipedia.org/wiki/Spherical_coordinate_system
Spherical coordinate system, Coordinate system conversions, Cartesian coordinates */
rxyz[i][0] = map(noise(rec+branches, i), 0, 1, 0, le);
an1 = map(noise(rec+branches, i+1000), 0, 1, PI/8, PI-PI/8);
an2 = map(noise(rec+branches, i+2000), 0, 1, PI/8, PI-PI/8);
rxyz[i][1] = rxyz[i][0] * sin(an1) * cos(an2);
rxyz[i][2] = rxyz[i-1][2] - rxyz[i][0] * sin(an1) * sin(an2);
rxyz[i][3] = rxyz[i][0] * cos(an1);
}
for (int i = 0; i < rxyz.length-1; i++) {
strokeWeight(1+rec);
line(rxyz[i][1], rxyz[i][2], rxyz[i][3], rxyz[i+1][1], rxyz[i+1][2], rxyz[i+1][3]);
drawBranch(rxyz[i+1][0]*0.8, rxyz[i+1][1], rxyz[i+1][2], rxyz[i+1][3], kn/2+1, rec);
}
popMatrix();
}
}
Comments
See the result here: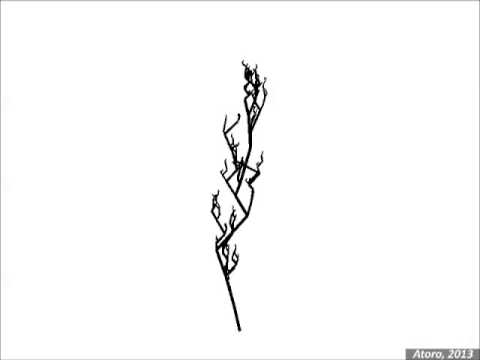
Nice !