ok guys, I got some of the program to work...
But, I can't get the line to draw properly. It draws like the figure below. It just move across like that. I want it to draw like a line graph...
Any help?????
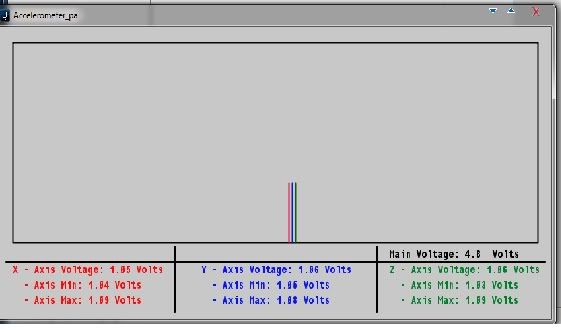
This is the processing code:
It's a bit messy, I haven;t cleaned it up yet...
Code:import processing.serial.*;
import processing.opengl.*;
Serial myPort; // The serial port:
int baudRate = 115200; //make sure this matches the baud rate in the arduino program.
int lf = 10; // ASCII linefeed
PFont myFont; // The display font:
int fontSize = 14;
float xAxisVal , yAxisVal , zAxisVal;
float sxAxisVal , syAxisVal , szAxisVal;
float xGForce , yGForce , zGForce;
float xAxisVol , yAxisVol , zAxisVol , mainVol;
float xAxisMinVol = 5 , xAxisMaxVol = 0 , yAxisMinVol = 5 , yAxisMaxVol = 0 , zAxisMinVol = 5 , zAxisMaxVol = 0;
float xVal , yVal , zVal;
float pyVal;
int xPos = 20;
int width = 820;
int height = 380;
void setup() {
size(width, height);
smooth();
// List all the available serial ports:
println(Serial.list());
// I know that the first port in the serial list on my machine
// is always my Arduino, so I open Serial.list()[0].
// Open whatever port is the one you're using.
myPort = new Serial(this, Serial.list()[1], baudRate);
myPort.bufferUntil(lf);
//set up the display text which will display the data recieved via serial
myFont = createFont("Ti73ProgCodeBold", fontSize);
textFont(myFont);
noLoop(); //don't redraw unless serial data so draw() will not loop.
}
void draw(){
smooth();
background(200); //nice light gray here
fill(#030000);
text("Main Voltage: " + mainVol , 580,300);
text(" Volts" , 725, 300);
fill(#FF0808);
text("X - Axis Voltage: " + xAxisVol + " Volts", 20,320);
text(" - Axis Min: " + xAxisMinVol + " Volts" ,20,340);
text(" - Axis Max: " + xAxisMaxVol + " Volts" ,20,360);
fill(#0513FF);
text("Y - Axis Voltage: " + yAxisVol + " Volts", 300,320);
text(" - Axis Min: " + yAxisMinVol + " Volts" ,300,340);
text(" - Axis Max: " + yAxisMaxVol + " Volts" ,300,360);
fill(#008127);
text("Z - Axis Voltage: " + zAxisVol + " Volts", 580,320);
text(" - Axis Min: " + zAxisMinVol + " Volts" ,580,340);
text(" - Axis Max: " + zAxisMaxVol + " Volts" ,580,360);
noFill();
tint(255, 128);
stroke(#050505);
strokeWeight(2.0);
rect(20,20, 780, 260);
stroke(#030303);
noFill();
smooth();
strokeWeight(3.0);
strokeJoin(ROUND);
beginShape();
line(10, 305, 810, 305);
line(560, 285, 560, 370);
line(260, 285, 260, 370);
endShape();
/*
stroke(255);
fill(#FFFCFC);
noFill();
smooth();
strokeWeight(1.0);
grid(20,20,780,260,10,#030303);
*/
stroke(#FF0303);
strokeWeight(2.0);
line(xPos , 280 , xPos , (260 - xVal));
pyVal=(260 - xVal);
stroke(#0017FF);
strokeWeight(2.0);
line(xPos + 5 , 280 , xPos + 5 , (260 - yVal));
stroke(#046400);
strokeWeight(2.0);
line(xPos + 10 , 280 , xPos + 10 , (260 - zVal));
if(xPos >= 780){
xPos=20;
}
else{
xPos++;
}
}
void grid(int x, int y, int w, int h, int n, color c) {
stroke(c);
for (int ix=x; ix <= x+w; ix += n) {
line(ix, y, ix, y+h);
}
for (int iy=y; iy <= y+h; iy += n) {
line(x, iy, x+w, iy);
}
}
void serialEvent(Serial myPort) {
// read the serial buffer:
String myString = myPort.readStringUntil('\n');
// if you got any bytes other than the linefeed:
if (myString != null) {
myString = trim(myString);
// split the string at the commas
// and convert the sections into integers:
float sensors[] = float(split(myString, ','));
xVal = map(sensors[0],0,1023,0,255);
yVal = map(sensors[2],0,1023,0,255);
zVal = map(sensors[4],0,1023,0,255);
mainVol = sensors[7];
xAxisVol = sensors[8];
if (sensors[8] < xAxisMinVol){
xAxisMinVol = sensors[8];
}
else if (sensors[8] > xAxisMaxVol){
xAxisMaxVol = sensors[8];
}
yAxisVol = sensors[9];
if (sensors[9] < yAxisMinVol){
yAxisMinVol = sensors[9];
}
else if (sensors[9] > yAxisMaxVol){
yAxisMaxVol = sensors[9];
}
zAxisVol = sensors[10];
if (sensors[10] < zAxisMinVol){
zAxisMinVol = sensors[10];
}
else if (sensors[10] > zAxisMaxVol){
zAxisMaxVol = sensors[10];
}
}
// add a linefeed after all the sensor values are printed:
redraw();
}