Hi,
I have a similar problem (I think) so I'm adding to this thread rather than creating a new one. For my own personal edification and enrichment, I'm making a clone of a flash game called gravity (link: http://adamatomic.com/gravity/ ). Right now I've succeeded in generating random mines (called "anchors") and spreading 6 of them throughout the stage. These anchors are each objects of a Class Anchor (basically a sprite).
Now I have a "rope" that is SUPPOSED to draw a line from itself to the first child of Anchor class "anc1" but for some reason it's not working. All I get is the below screenshot. Looking at my code, it seems that there are some serious redundancy issues, but I don't know how to solve it. Some help would be great. I have also since ordered the orange-colored processing book off Amazon.
Code:
//Class Anchor has 6 children.
Anchor anc1;
Anchor anc2;
Anchor anc3;
Anchor anc4;
Anchor anc5;
Anchor anc6;
Rope rp; // A single line that draws from nearest anchor to mouse.
PImage anchor; //We have a sprite that will be implemented later.
float xpos;
float ypos; //I am unsure if I need these.
void setup()
{
size(240,480);
background (110, 110, 120);
stroke(25,30,0);
line (0, 470, 240, 470); //this is the "ground"
noLoop(); //if this is removed, the program will never stop adding anchors.
}
void draw()
{
xpos = random(10,200);
ypos = random(20,440); //generate random location.
anc1 = new Anchor(xpos, ypos); //puts a new anchor at this location.
anc1.display (); //anc1 child uses display function.
rp = new Rope(xpos, ypos, mouseX, mouseY); // New rope? it should draw from anc1 to the mouse.
rp.display (); //display the rope.
xpos = random(10,200);
ypos = random(20,440);
anc2 = new Anchor(xpos, ypos);
anc2.display ();
xpos = random(10,200);
ypos = random(20,440);
anc3 = new Anchor(xpos, ypos);
anc3.display ();
xpos = random(10,200);
ypos = random(20,440);
anc4 = new Anchor(xpos, ypos);
anc4.display ();
xpos = random(10,200);
ypos = random(20,440);
anc5 = new Anchor(xpos, ypos);
anc5.display ();
xpos = random(10,200);
ypos = random(20,440);
anc6 = new Anchor(xpos, ypos);
anc6.display ();
}
class Anchor {
float x, y;
Anchor (float xpos, float ypos) {
x = xpos;
y = ypos;
}
void display () {
anchor = loadImage("anchorgrn.gif");
image (anchor, xpos, ypos);
}
}
class Rope {
float x1, y1, x2, y2;
Rope (float x1, float y1, float x2, float y2) {
x1 = anc1.x;
y1 = anc1.y;
x2 = mouseX;
y2 = mouseY;
}
void display () {
stroke (200);
line(x1, y1, 50, 100);
println(y1);
}
}
results:
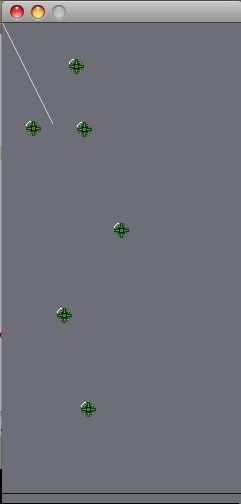
On earlier builds, the rope wouldn't draw at all, which is why I inserted constants in the display function. Anyways, some pointers would be great.
(i hope php tags work)