We are about to switch to a new forum software. Until then we have removed the registration on this forum.
Are there any known issues that one needs to always keep in mind when trying to port code from GLSL Sandbox to Processing?
I'm trying to get this fragment shader code to work (in a file 'frag.glsl'):
http://glslsandbox.com/e#39373.0
#ifdef GL_ES
precision mediump float;
#endif
#extension GL_OES_standard_derivatives : enable
uniform float time;
uniform vec2 mouse;
uniform vec2 resolution;
void main(void){
vec2 p = (gl_FragCoord.xy * 2.0 - resolution) / min(resolution.x, resolution.y);
vec3 destColor = vec3(0.0);
for(float i = 0.0; i < 200.0; i++) {
float j = i + 1.0;
vec2 q = p + vec2(cos(j), sin(time * j)) * 0.5;
destColor += 0.0004 / length(q);
}
gl_FragColor = vec4(destColor, 1.0);
}
in the following sketch:
PShader basicShader;
void setup(){
size(400,400, P3D);
basicShader = loadShader("frag.glsl");
basicShader.set("resolution", float(width), float(height));
}
void draw(){
basicShader.set("time", millis() / 1000.0);
shader(basicShader);
rect(0,0,width,height);
}
And instead of producing loads of white points, the Processing sketch produces only two.
Is there something particularly different about the variables that are passed to the fragment shader in Processing compared to the GLSL ones?
Thanks.
Answers
This works for me.
It seems to be the case that very subtle things such as what you name your variables.
If you need a "buffer" (not needed in your case) you need to do it manually, while it works automatically in sandbox.
Other than that annoyance it seems like there are more features that work in processing than in sandbox.
Huh... that's weird. It looks like you didn't need to change the code?
I copy-pasted your code in to be sure and its still just two points moving up and down rather than the animation online...
Are you on Processing 3.3?
@smokeredsky

yes, and it's many poin't and flashes moving just fine
Far out. Think I'll have to leave this one till I know more about the entire pipeline to figure it out...
Thanks for checking this. Much appreciated.
processing registers some variables with obvious names and it's easy to accidentally duplicate these, which causes problems.
here's a block of code that references shader variables. i'm not sure it's the complete set
https://github.com/processing/processing/blob/master/core/src/processing/opengl/PShader.java#L1120
https://github.com/processing/processing/blob/master/core/src/processing/opengl/PShader.java#L1146
probably best to avoid these names.
@koogs, nice finding
ambient
color
direction
emissive
lightAmbient
lightCount
lightDiffuse
lightFalloff
lightNormal
lightPosition
lightSpecular
lightSpot
modelview
modelviewMatrix
normal
normalMatrix
offset
perspective
position
ppixels
projection
resolution
scale
shininess
specular
texCoord
texMap
texMatrix
texOffset
texture
transform
transformMatrix
vertex
viewport
Thanks all. I've changed the resolution and time variables to u_resolution and u_time but its still only two points.
Will update if I find a fix that's useful to others somehow...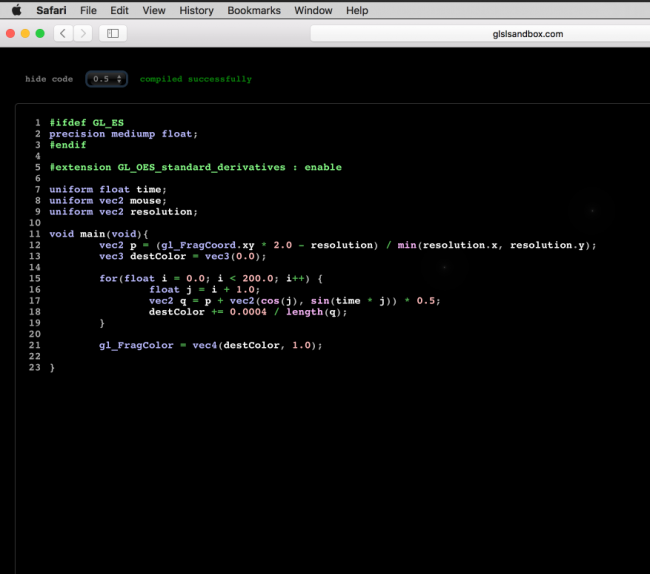
I think it's something to do with this particular shader as others from GLSL Sandbox work fine. That variable set in the sandbox that goes from 0.5 - 8 seems to change the amount of points but its not accessed in the fragment shader itself.
Also - the 'two point' version is also what happens when running the sandbox code in Safari (you can barely see the points in this screenshot )
please don't post screenshots of code. they are infinitely less useful than code we can cut and paste and run ourselves.
also, that screenshot doesn't show the changed variables.
That screenshot is of the GLSL sandbox code running on the site as is on Safari to demonstrate that the issue is not isolated to Processing itself for this particular shader.
The two points are barely visible but they are inline with line 9 and 14.
Still not very useful in solving the problem of why this shader code runs like that in Processing on my system though..
Changing the loop variable to int may help:
Yes, that worked. That is kind of weird though.
When using floats in the for-loop, it terminates at 2. The sketch works the same if its an int with i<2 as the condition as it does with a float with a condition of i<n, where n is any number above 2.
For some reason, Processing's implementation doesn't like floats in for-loops maybe?
I've seen the same thing with for loops and floats, but only on certain systems / drivers - OSX and Intel IIRC. I don't think it's specific to Processing.