We are about to switch to a new forum software. Until then we have removed the registration on this forum.
Using this code to toggle modes between on and off:
if (keyPressed && key == 'm')
{
if (menu)
{
menu = false;
}
else
{
menu = true;
}
}
is fine in theory. However the result flickers and the out come isn't always the desired one. I compare this to like debouncing physical buttons in arduino, however I'm a little lost this being processing and not using buttons, or having delay.
Thank you in advances! :)
Answers
when this is part of draw() it gets checked 60x per sec - therefore flickers
better:
own func, gets only to run once every key press
it's an in-build func, see reference
short
menu = !menu;
edit: ops, late...
That's probably because you are checking for keyPressed in draw 60 times each second. So It keeps changing between both states. I would use
keyPressed
the function, orkeyReleased()
so it won't meter how long user hold the key pressed. Also there is the notationmenu = !menu
that will toggle the state of the boolean.untested
that should do it ; )
edit: it seems I was even later, at least everyone was thinking of similar solutions
The problem is that this code is probably in your draw() loop and is thus run 60 times per second. The outcome is unpredictable as it will just go back and forth many times.
The solution is to move this code to the keyPressed() method external to draw(). Also there is a shorter way to code 'invert the boolean variable'. Adapted code:
just for once I was the fastest...
but I still feel this is not well documented and hard to find
Wow thank you all for these answers! It will certainly help me from now on and I really appreciate it! Hopefully this will help other people with this issue. And again thanks!! :)
Oh wait... I can only do it once! NONONONONONONO!!!!!
It says: dublicate method keyPressed() in
WHAT DO I DO!!! I ALREADY ACCEPTED ANSWERS!!!!! NNOOOOOOO
Ahhh this kinda sucks. I should go check the function refference.
We can't have methods sharing the same name within the same class.
If you need keyPressed() callback method to check more things out, just use more
if ()
&else if ()
blocks there! ;;)Crazy compact: menu=key=='m'?menu:!menu;
The code should look like:
If you can get it working, consider posting a sample code that produces the error
Ohhhhhhhhhh... I see. So somehow for some reason when the true is returned from keypressed that activates the function? And then from there I can put more into it. AWESOME :)
I got it working, thanks everybody, however other parts of my program are failing. Such as for whatever reason to get into fantom mode I must be in another mode first... I don't know why that is, and I can't get the light black part to cycle nicely. I'll work on that later but that if else needing another one activating first is stumping me. And some weird bugs are happening where the ball just goes through the wall and you have to watch it in action but if you's guys have any suggestions or notices or tips 'n' tricks it would appreciated, and help me learn tremendously. This is after all only my second 'real' program (asides from a couple number guessing console games in C++ and what have you with arduino).
Here is my program:
Hello,
you wrote
I don't see - I can press f at start and be in fantom mode (dark gray) immediately.
What do you mean?
Anyway
sometimes it's worth to re-write your code a little
it gives you practice
first I'd suggest to put all key related stuff into your new function
you can start there by saying
It's best to have all key-stuff in one place
Next
ok, then I suggest you make more functions like:
etc.
that gives you practice
So take a bunch of lines and put them out of draw() in an own function and give it a good name (and write the name of the func into draw() where the bunch of lines was before of course)
please do a lot of testing after a change to make sure it still works
draw() could contain then only managaPaddle, drawBall etc., so it is very readable then
Also
At the moment, you add the same value to ball x and y.
so it can only at 45° - which is boring
instead you can have float ballAddX and ballAddY and add them
Also I noticed a stuttering when ball is close at paddle. Bad. You can avoid that when you say
Greetings, Chrisir
I just tried out my sketch again and Fantom (Yes Google, I know it's spelled 'phantom', put I need p for psychedelic, phantom and players so I changed it. No squiggly red line needed) mode worked immediately. Odd considering it wasn't the other day.
Anywho
ballAddX & ballAddY, do mean like make those a function and then call them when needed? Or they are like PPF (Pixels Per Second) but for X and Y both, not just a direction? I am a little unsure of what you mean.
Also I will never use somebody else's code unless I understand it (just how I am, I feel it to be cheaty and has the possibility to cause problems later on - because I don't know how it works, I can't fix it) and I don't quite understand what abs()ing it will achieve? I'm guessing that ballAddX is what is making it go sideways and turning it positive will immediately make it head the other direction? Or something of the sort. But also what will happen when when it reaches 32 PPF (what I will cap it to). If it's right at the edge, the moves 32 pixels, it will completely bypass it. Currently I'm pretty sure this already happens with the edges, but the change in direction quickly puts it back. I'd love to know how these type of this get fixed, not so much for my program to work, but because I'm curious and excited to know how that protocol will work =D
And thank you for your tips. if (key == CODED) ect. I will definitively be using that from now on, it is genius! For the draw(), I will add them as functions, and luckily the "chunks" of code to go in them are mostly already laid out for me ;)
I do have a question though about functions, I was going to make a thread but perhaps here will do. How exactly do the functions, draw, and other functions (void keypressed) all work? I understand draw is the infinite loop that runs so long as the program is open, and that for example "int fucntionName(X, Y, Z) { }" is placed outside the loop and is then called for when your using it multiple times or to conserve space in (tidy up) draw(). But what about the void keyPressed type ones. Do they run like a separate draw? How are they activated exactly? They don't run at 60x per second, but only when keyPressed is true, but does it check for that every 60x per second too? What am I missing here? I couldn't find anything (exept for string functions) in the reference.
Thank-you all very much!
Actually, testing for CODED is completely unnecessary within callbacks! L-)
Here's an online example which uses keyCode only and got no need to test for lower case at all:
http://studio.processingtogether.com/sp/pad/export/ro.9bY07S95k2C2a/latest
PApplet.main()
Well, that's a short description of what I investigated till now! :-B
@ GoToLoop : I still think if (key == CODED) is useful in keyPressed() because you structure it in two parts and can find your entries (F1 oder f...) more easily
@ MadScience2728 : ballAddX & ballAddY are both global vars.
instead of
say
instead of having directI(), directII() etc. you only have move()
but ballAddX & ballAddY can both have neg values.
Look a the examples
http://processing.org/examples/bounce.html
(here the bouncing is different than I'd do it)
for stuff like abs looks at the reference
http://processing.org/reference/
It seems 1 more slow layer to check against! That would be useful only if we had 2 big
if
CODED & !CODED blocks.Or even better, if we're not interested in 1 group, just abort keyPressed() right way:
Most of the time we're not interested in discriminating between lower & upper letter cases!
Therefore, variable key is completely unnecessary!
By using keyCode alone, we can check all available keys, no matter if they're CODED or not!
With the added bonus of not worrying about letter cases either! \m/
My issue about
if (key == CODED)
is that it is taught as if it was an obligatory step! ~X(As if the code would be somewhat flawed or bugged otherwise! Spreading like superstition to every1! :^o
Looks to me as if Chrisirs way would be for more noobies, and then when they get a little more comfortable go with Loops way. Both good in my opinion, I guess it'll just depend on your situation and what not.
Anyway thank you's guys tremendously! I will change the directIs to a move function and base it off of the reference sketch linked. This will make implementing angles more than 45d much easier!
@ MadScience
in the example it says when you hit left / right wall: just ballAddX = -1 * ballAddX /// meaning make a pos number neg or a neg number pos
same for upper and lower wall
but this can lead to stutter and that's what we see in your sketch
so I keep saying:
check left and right wall seprately
on left side say ballAddX = abs (ballAddX); /// means it gets always pos
on right side say ballAddX = -1 * abs (ballAddX); /// means it gets always neg
@ gotoloop
I agree it's not mandatory but when you have long keyPressed with mixed checks for 'a' 'b' F1 F1 UP DOWN etc.... two big if CODED & !CODED blocks structure it better
like this:
I thought my example showed that I've cached keyCode as local variable k? 8-}
I hate typing long names. I'm lazy! :!! Also, local variables are faster than field 1s! \m/
And as shown, I mix both CODED & !CODED together: :ar!
I've mentioned that as a valid case for splitting them:
"That would be useful only if we had 2 big if CODED & !CODED blocks."
@goToLoop indeed I liked learning that the check to see if "key is coded" is not mandatory, thanks ;)
Well I made the conversion from direct to ballAdd. I'm still unsure what will influence the change in rise/run (ballAddY, ballAddX) yet but I'll get there.
So here is the sketch so far but I'm having troubles making the ball increase speed like before. I tried adding PPF to ballAddX/Y but that failed. Then I added it right in the ballX/Y = ballAddX/Y ; and that didn't work either. Then I multiplied ballAddX/Y by PPF to see if it would keep the negative aspect and it just freaked out.
Here is the code: Any thoughts?
Well this seemed to fix it but now it does not turn the value negative. I'm gonna go test to see if it's still detecting that it passed or not.
I think it's about time I move this into the 'My Projects' section.
Figured it out!
Set ballAdds to 1 so they only change direction, then we multiply PPF by them so that were using PPF again for ballX & ballY but now their polarity is based off of ballAdd. So really ball add is now ball direct, and PPF will be need to have of each for X and Y and it really will ballAddX/Y soo.... Not too confusing I guess.
Congratulations!
I feel your program is better now...
draw() looks very clean
It's "boundaries" btw with a
Does the menu work?
additional cleaning up
Maybe you can additional cleaning up:
e.g.
I suggest getting rid of "direct" altogether (can you? I dunno)
But I think direct is replaced by ballAddX and ballAddY, isn't it?
in the function randomStart() we want to give ballAddX and ballAddY random values!
or so - only then you achieve different angles in which the ball flies...
Good work!
Greetings, Chrisir
;-)
I have more ideas in store for you but step by step....
:D
I thought I had gotten rid of all the directs? Oh well I'll go back and make sure, because yes it has ;) I haven't yet re-wrote the random start so that's why it's like that, I'll add it to the to-do list, as well as changing boundries.
Ok control F'd and replaced all boundries with boundaries =D And rewrite the random is added to to-do.
Also menu isn't operational yet (And from my brief testing it out it didn't work) so I should be heading off to bed but I mean come on, coding or sleep, what would you choose lol :P.
Ok I'm off to test menu, but first I want to say thanks so much ppls! Especially you chrisir, your getting a thanks on the bottom of the menu or something ;) So I'll (try) to get menu up, but meanwhile by all means chrisir suggest your ideas, I'm all ears. 2 ears. Actually 2 eyes. Cuz you know, text and all. I have a to-do list with Main Task, Secondary Task, Eventually Task, and Bugs Task, and I can add them there.
Ok the code is here: https://www.dropbox.com/s/ptanuzei3v9bjct/Pong_BETA_V1_2_3.pde
///////////////
Also why I was here in the first place. Do you's guys know exactly why the ball was studering so much around the paddles and occasional wall. I know PPF would have been a problem making it skip right over the line in one frame, but then it would change direct and then problem solved. But if I recall that wasn't always the case, and it would stray way out then come back in. And when it would get stuck "inside" the paddles, that just confused me since the paddle was in essentially 1d relative to how the ball could hit it so there was no "inside". My guess is that when it would be near, the speed of it (PPF) would jump it right through, then the direction would change, it would go out of it, and for some reason it would change back and repeat, hitting the paddles again.
Sorry if this is all worded a little funny I'm tired.
Yeha menu isn't really going well and I'm stumped for now..
It's getting stuck in menu, and unresponsize to the menu commands when in the while loop. And the screen is white.
See I need it to be in an infinite loop right there so long as menu i
I'm going to bed now.
you wrote:
That's easy.
you check whether the ball is in a zone at the paddle / near wall
if so, you changed direct and substracted PPF
good.
but now in the next loop of draw() the ball is still in the zone: you again change the direct (although it's correct already), so we add PPF again so it goes deeper into the zone, stays in the zone...
and therefore it stutters...
My additional ideas
the menu problem:
in draw() have a switch(state) { } and have for each state one paragraph
in keyPressed also have
when user hits the correct key in state menu say
yes, you can give the states names, e.g. stateGame
to demonstrate states with a menu...
also in mousePressed you want to use state
as you can see, draw() has gotten long again -
you could again kick out splash screen as an extra function....
Ok that's just a wee bit complicated... I'm working my way through it. It seems pretty straight forward if you ignore the visual stuff. In the draw() put a switch state. Based on the switch state it will run different screens. And as for the void keyPressed I finally got that. Realizing that oh yeah, this is keyPressed helped. Cool. I will certainly implement this. And basically it will have one state for play game, and that's where my game play functions will be right.
Good idea for how I can implement angles changing. But then if someone hits it on the end, then it could go all very narrow zig-zag or some other weird angle. Do you have any suggestions on ways to implement angles? After reading this it did spawn the idea to have the end thirds of the paddles at 22.5 degrees. Then change angle based on that. But that would mean figuring out when the ball hit it and sigh. I'm post boning different angles to the eventually section for now. PLEASE HELP WITH ANGLES!!!! I'm gonna go play pong games to see what they did for angles :D
Ahh great idea for a like a I can't remember what they're called. But yeah, I could use switch case to bring it into that 'Mode' and then I could call the regular functions but add the ones for the walls. I think programming it will be funner than the mode itself. And then the moving walls thing! But what are they called. It's not gonna be game modes because that's already taken. But you know theres classic, walls, super speed, hard mode etc... Wow this is givng me the idea to but the colour modes in the menu and man! This is gonna be awesome! My menu will have settings! I'm exited!
Um bricks? Like moving obstacles? Thought about, forgot about it. I will be adding that to the list. I could use sin/cos so that it's not just linear movement up and down, and yeah I like it.
Levels? I kinda have that, I have PPF ball speed. It goes up to 25 Pixels Per Frame and slowly increases. And then with the colour modes I think this is it?
Sorry no. Were can I find him. Is he youtube or forums or other?
Well cool thanks for the suggestions, I will implement menu then using the switch case I can put in different options like an options menu and coulour modes that you turn on. It'll be great!
Damn angles... My other option asides from 45Degrees is to invert the angles.
Like if the ball its the wall C at 10 degrees heading for D, it leaves at 170 degrees. Or hits heading for D at 100 degrees, hits A, then leaves it at 80 degrees. If you know what I mean.
What do you think?
The angle is just a result of balladdx and balladdy
The angle is just where the ball goes because of the two
Angle is not a var in your sketch!!
Sorry.
Anyway at upper edge of right paddle just say
Balladdy -= 1;
So it goes a little more sideways than in the middle of the paddle
When both are same angle is 45
When its 1 and 2 angle is e.g. 22.5
Just remember that ballAddX and ballAddY are changed to ballDirectX/Y. They are also set to 1, and 1 to remain. As we multiply PPFX and PPFY by them it changes the direction. So now ballAddX/Y are PPFX/Y (Pixels Per Second X/Y) and by changing these it changes the angle (rise/run (PPFY/PPFX)).
And no I know :P But my question is how will I determine angle change based off of when it hits. For example right now it hits at 45d then leaves at 45d (1/1). Should I make it so that if it hits at 2/1 should I change it -1/2 (perpendicular angle)? Or should I make it -2/1 (Inverted angle, other example: 2/4 → -2/4). Or some other suggestion?
Also, but then what factors will change the angle? What will make it go from 1/1 to 2/1 which then I can hit against a wall and then -1/2 or -2/1 it. Because other wise if it's at default 1/2 it will always only be some variation of that. The thing I see most pong games do is something I don't know how to do. They make it so when the ball hits the paddle, if the paddle is moving then alter the angle based of that.
Like if the ball is on trajectory -1/2, hits the paddle, and instead of going back at 2/1 (perpendicular), if the paddle is moving up then come back at 1/4.
I don't know exactly how that would work. Like I'd have to check the difference in position of the paddle, then apply that to the angle. So I'll do that after the menu :/
Idea!
I should set a level thing and call it Super Random! Basically almost every int/float in the game gets a random value between 0, and twice it's default! And booleans too.
the idea
red normal reflect
yellow - stronger reflect, that would appear on the edge of the paddle at the lower side
think of it has the edge of the paddle a little rounded
so when he hits on the broad side, normal reflect
when he's near edge, have a formula the closer to the edge, the bigger balladdy gets (+0.1, then +0.2....)
it's only a gimmick
also when the paddle has a speed you can also change the direction of the ball when he hits it
An good idea and I could just make it invisible so the user doesn't notice and then maybe even do it with the sides.
As for the paddle speed thing I've been wanting to do that all along as I always see that in these type of games, however I'm not sure how to do it.
Chrisir, what do you know about xbee wireless, and serial data via processing? I just got a rover in the mail and will be really getting into serial communication soon so I can command the rover via a emotiv mind reading headset, into processing (like a wasd type thing) then sending that to an arduino, then wirelessly to the robot. A.k.a wirelessly controlling a robot to move around with my thoughts alone.
The graph post I made, that's actually because in the live data feed window, I want to have graphs reporting back on board accelerometer data. And the pong game never meant to go so huge as it is. It was honestly just supposed to be a nice small pong game just to be like wow, I was able to make a pong game! Because asides from like testing things it was my second real program.
Anyway I want to say thanks to all you's guys whom for without I would not be where I am =D
hi!
sure the pong s only for training - forget about it.
Your new project sounds absolutely cool and I know nothing about it
so it's worth starting a new thread...
Greetings, Chrisir
I won't be yet unless I need some help getting xbee and arduino and processing communicating, and I don't have the headset yet. It's 300 bucks and I don't have a job, so I'm practicing getting really good at coding the other parts first :P
As for pong I figure I'll finish the menu, then leave it for when I'm older and an expert programmer and be like (in 30 some year old me's voice): "Oh yeah, I remember these old projects I never finished, I think I should finish them finally". Only to rewrite the entire codes thinking how crappy I was back then lol.
Pong was a great start and it taught me alot. My next big project (solo processing i.e. no mind reading) I'm hoping to be a evolution simulator of sorts. Basically have a box, with 3 legs, and 3 fore legs attached to them. There will be 12 variables, 6 for speed, and 6 for how far each one can turn. 12 will be spawned. The movements will be random at first, but the two that make it the furthers will 'mate' and the variables for their motion mixed up and mutated slightly, creating 12 offspring. Then the two of them that go furthest... And repeat.
I know you can hinge one part of another, and I know I can apply basic gravity, and make sure certain parts don't touch the ground. But that't about it.... Making it move as a whole thing, well I cross that bridge when I get there. Gulp. I imagine it's a big bridge....
Cheers
Mad Science
there are headsets at 10 to 30 bucks
Not like this one. This one you train. You start by given it nothing. It reads your brainwaves for 8 seconds, while you think of nothing. Then the fun begins. There is a vitual cube on the screen. And you train the software to move it with your mind. Example: for 8 seconds you think "move forward" or something. And you repeat this several times for different things like rotation, move left, right, up, down forward back ect. Then you set it so control keys on your keyboard.
Heres where processing comes in. I detect those keys, then send them serially to the arduino. Then from there wirelessly to the rover.
And that is my plan! Here are some links to the headset:
Ted Talks: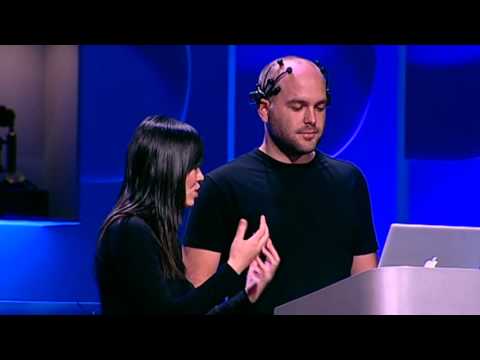
This youtube dudes video that convinced me this is the right one: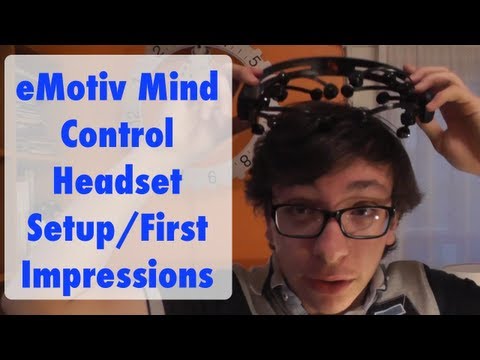
The website for it: http://emotiv.com/
A 10 - 30 dollar one will not suffice unless I attach it to one of those crappy RC cars that only go forward and back. Which I'll probably do for my little bro since hes got one.
But with this headset, I can also make a robotic arm, attach it to a quadriplegic (or Stephen Hawking) and give them an arm per-say.