We are about to switch to a new forum software. Until then we have removed the registration on this forum.
Hi, I've tried to make a program that shows a 2D image in 3D, using the brightness of each pixel. So I draw a box at each x and y, the z is the birghtness. Unfortunately, the only way I've found to see the image is to draw boxes in draw(), but it gets really slow for images bigger than 400*400. I think that there are ways to make that better, so how can I do it ?
Code :
import peasy.*;
import peasy.org.apache.commons.math.*;
import peasy.org.apache.commons.math.geometry.*;
PeasyCam camera;
PImage img;
void setup() {
size(800, 800, P3D);
ambientLight(255, 255, 255, 0, 0, 0);
img = loadImage("download.jpg");
camera = new PeasyCam(this, img.width/2, img.height/2, 0, 1000);
img.loadPixels();
}
void draw() {
background(0);
for (int i=0; i<img.pixels.length; i++) {
color c = img.pixels[i];
float b = map(brightness(c), 0, 255, -100, 100);
fill(c);
noStroke();
int x = i%(img.width);
int y = (i-x)/img.width;
translate(x, y, b);
box(1);
translate(-x, -y, -b);
}
}
Answers
640,000 boxes...
The geometry is static so try a PShape. Also, boxes that small probable won't look like boxes so try points instead - no need to draw 6 sides per pixel that way.
But the main problem is the sheer number of boxes you're trying to draw
I can draw less boxes, but I'll lose resolution. And I can't get the PShape method to work :
can't test the code at the moment but that translate at line 24 looks like it needs applying to the part. otherwise where are you specifying the position of the cube within the group?
there is a limit to the amount of things you can put into a shape, depends on your graphics cards. i also find that adding things as children incurs some overhead, as if something iterates through the children one at a time. but you have no choice here, i don't think (unless you want to add your own quads to a single shape, 6 for each cube)
In your first solution : you could fill an ArrayList of boxes in setup and display the ArrayList
But your 2nd solution without line 30 And some corrections looks great
example for solution 1 with ArrayList holding boxes of type class Box (another topic but same class and similar filling of the class in setup / generate):
Thank you but with that, I still get low framerate. I've tried with triangles strip and it works great :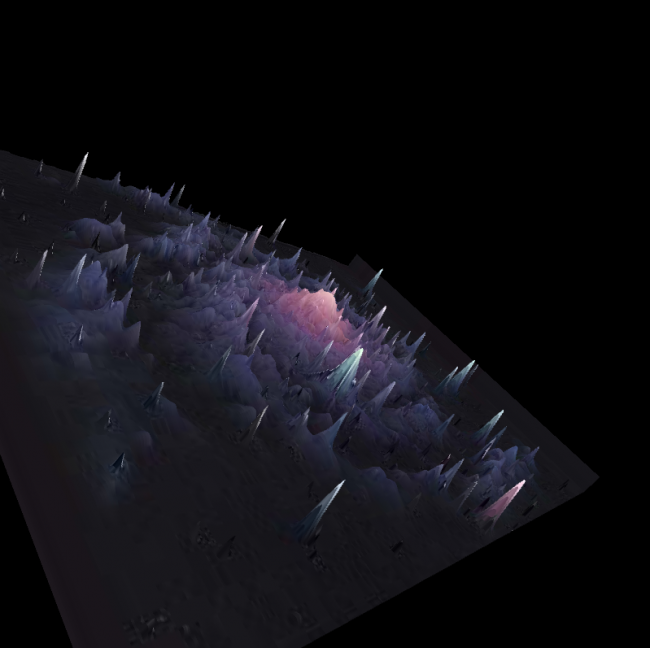
Tell me if there is some adjustments/optimizations to be made :
Not possible to store what draw does in a PShape and display that?