We are about to switch to a new forum software. Until then we have removed the registration on this forum.
I'm trying to practice using matter.js to create top down levels Bomberman/Zelda style.
Right now I want to get my circle, which is controlled by arrow keys to move and bump into static squares but it is just going through them. Did I set it up incorrectly? I have been coding for three months, so I might be quite slow sorry!
var Engine = Matter.Engine,
World = Matter.World,
Bodies = Matter.Bodies;
var engine = Engine.create();
var world = engine.world;
var player;
var rocks = [];
var cols = 7;
var rows = 7;
function setup() {
createCanvas(750, 750);
Engine.run(engine);
player = new Player(300, 300, 25);
var spacing = width / cols;
for (var j = 0; j < rows; j++) {
for (var i = 0; i < cols; i++) {
var r = new Rocks(i * spacing, j * spacing);
rocks.push(r);
}
}
}
function draw() {
background(51);
Engine.update(engine);
for (var i = 0; i < rocks.length; i++) {
rocks[i].show();
}
player.show();
player.move();
}
function Player(x, y, r) {
this.body = Bodies.circle(x, y, r);
this.r = r;
World.add(world, this.body);
this.show = function () {
ellipse(x, y, this.r * 2);
}
this.move = function () {
if (keyIsDown(RIGHT_ARROW))
x += 10;
if (keyIsDown(LEFT_ARROW))
x -= 10;
if (keyIsDown(UP_ARROW))
y -= 10;
if (keyIsDown(DOWN_ARROW))
y += 10;
x = constrain(x, this.r, height - this.r);
y = constrain(y, this.r, width - this.r);
}
}
function Rocks(x, y, w, h, options) {
var options = {
isStatic: true
}
this.body = Bodies.rectangle(x, y, h, w, options);
this.w = w;
this.h = h;
this.size = player.r * 2;
World.add(world, this.body);
this.show = function () {
rect(x, y, this.size, this.size);
}
}
Answers
I don't see in your code where you check for figure overlapping. One way to do this is to add a new function to Rocks that check for collisions, like this:
For collision algorithms, check previous posts or the link below:
https://forum.processing.org/two/search?Search=collision
http://www.jeffreythompson.org/collision-detection/table_of_contents.php
One thing you need to keep in mind is if your player stepping is bigger than the dimension of your block, you could be missing it so you need to keep in mind if you change the size of your rocks.
Kf
Hi,
Yeah I was hoping that the physics engine Matter.js would handle the collision.
I am aware of how to do this "manually" per se. Thanks for the help tho, I'll keep this updated once I find out why the engine isn't working.
@pyan83 I can see my post is not relevant to your discussion. I am not familiar with the Matter.js engine. Hopefully you can figure what is missing in your code (I guess you are watching shiffman's videos?) and super awesome that you share your answer here.
http://brm.io/matter-js/
Shiffman in action: Physics engine update:
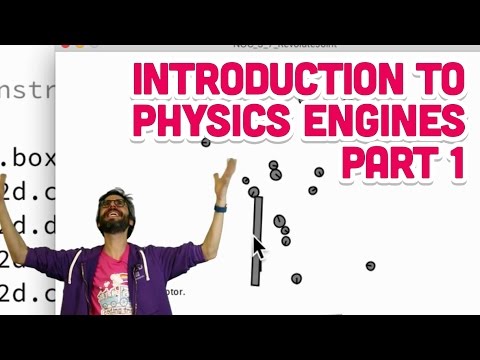
Kf
I think you have an issue where you are controlling xy positions rather than letting the physics engine handle those positions and querying the engine in order to draw stuff.