We are about to switch to a new forum software. Until then we have removed the registration on this forum.
There's a nice canvas effect on this website (waaark.com) When you mouse over the different sections there's a wobbly wave effect. Could you make this using p5 and if so how would one go about it?
Answers
This is about as far as I got trying to replicate it. Not sure why the colors flicker wrong. Maybe someone else can take it from here.
I don't think I explained well enough here. I meant once the page has loaded and there three different coloured sections. when you pass the cursor through the different sections, their bounding box wobbles like a jelly effect. You can see below in the image.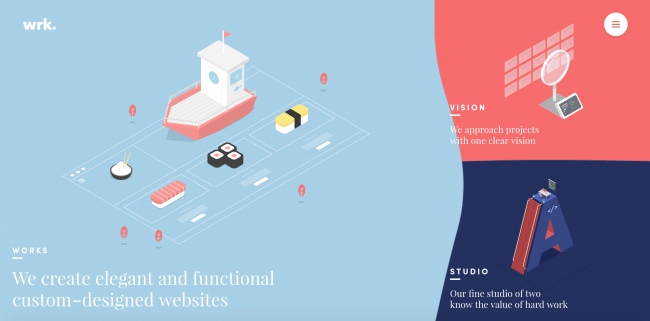
Great effect @TfGuy44. Thxs for sharing it (A+ for effort and intentions!)
Kf
it also makes my CPU go crazy, solid 100% utilisation of one of the cores.
Sorry, I'd also like to say thanks @TfGuy44 for your effort and intentions. Also I'm not bothered about optimisation or anything, I'd just like to know a way of obtaining this or any similar interactive effect for educational purposes. Doesn't have to be exact I'd just like to learn the principles because I don't even know where I would start at this point. Thanks.
Check this: http://p5js.sketchpad.cc/Pj6Y0NTGJl
Kf